#include <ESP8266WiFi.h> //https://github.com/esp8266/Arduino
//needed for library
#include <DNSServer.h>
#include <ESP8266WebServer.h>
#include <WiFiManager.h> //https://github.com/tzapu/WiFiManager
void setup() {
// put your setup code here, to run once:
Serial.begin(115200);
//WiFiManager
//Local intialization. Once its business is done, there is no need to keep it around
WiFiManager wifiManager;
//reset saved settings
//wifiManager.resetSettings();
//set custom ip for portal
//wifiManager.setAPStaticIPConfig(IPAddress(10,0,1,1), IPAddress(10,0,1,1), IPAddress(255,255,255,0));
//fetches ssid and pass from eeprom and tries to connect
//if it does not connect it starts an access point with the specified name
//here "AutoConnectAP"
//and goes into a blocking loop awaiting configuration
wifiManager.autoConnect("AutoConnectAP");
//or use this for auto generated name ESP + ChipID
//wifiManager.autoConnect();
//if you get here you have connected to the WiFi
Serial.println("connected...yeey :)");
}
void loop() {
// put your main code here, to run repeatedly:
}
ARDUINO Scrolling Text on 8x8x2 LED MATRIX
#include <MaxMatrix.h>
#include <avr/pgmspace.h>
PROGMEM const unsigned char CH[] = {
3, 8, B00000000, B00000000, B00000000, B00000000, B00000000, // space
1, 8, B01011111, B00000000, B00000000, B00000000, B00000000, // !
3, 8, B00000011, B00000000, B00000011, B00000000, B00000000, // "
5, 8, B00010100, B00111110, B00010100, B00111110, B00010100, // #
4, 8, B00100100, B01101010, B00101011, B00010010, B00000000, // $
5, 8, B01100011, B00010011, B00001000, B01100100, B01100011, // %
5, 8, B00110110, B01001001, B01010110, B00100000, B01010000, // &
1, 8, B00000011, B00000000, B00000000, B00000000, B00000000, // '
3, 8, B00011100, B00100010, B01000001, B00000000, B00000000, // (
3, 8, B01000001, B00100010, B00011100, B00000000, B00000000, // )
5, 8, B00101000, B00011000, B00001110, B00011000, B00101000, // *
5, 8, B00001000, B00001000, B00111110, B00001000, B00001000, // +
2, 8, B10110000, B01110000, B00000000, B00000000, B00000000, // ,
4, 8, B00001000, B00001000, B00001000, B00001000, B00000000, // -
2, 8, B01100000, B01100000, B00000000, B00000000, B00000000, // .
4, 8, B01100000, B00011000, B00000110, B00000001, B00000000, // /
4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // 0
3, 8, B01000010, B01111111, B01000000, B00000000, B00000000, // 1
4, 8, B01100010, B01010001, B01001001, B01000110, B00000000, // 2
4, 8, B00100010, B01000001, B01001001, B00110110, B00000000, // 3
4, 8, B00011000, B00010100, B00010010, B01111111, B00000000, // 4
4, 8, B00100111, B01000101, B01000101, B00111001, B00000000, // 5
4, 8, B00111110, B01001001, B01001001, B00110000, B00000000, // 6
4, 8, B01100001, B00010001, B00001001, B00000111, B00000000, // 7
4, 8, B00110110, B01001001, B01001001, B00110110, B00000000, // 8
4, 8, B00000110, B01001001, B01001001, B00111110, B00000000, // 9
2, 8, B01010000, B00000000, B00000000, B00000000, B00000000, // :
2, 8, B10000000, B01010000, B00000000, B00000000, B00000000, // ;
3, 8, B00010000, B00101000, B01000100, B00000000, B00000000, // <
3, 8, B00010100, B00010100, B00010100, B00000000, B00000000, // =
3, 8, B01000100, B00101000, B00010000, B00000000, B00000000, // >
4, 8, B00000010, B01011001, B00001001, B00000110, B00000000, // ?
5, 8, B00111110, B01001001, B01010101, B01011101, B00001110, // @
4, 8, B01111110, B00010001, B00010001, B01111110, B00000000, // A
4, 8, B01111111, B01001001, B01001001, B00110110, B00000000, // B
4, 8, B00111110, B01000001, B01000001, B00100010, B00000000, // C
4, 8, B01111111, B01000001, B01000001, B00111110, B00000000, // D
4, 8, B01111111, B01001001, B01001001, B01000001, B00000000, // E
4, 8, B01111111, B00001001, B00001001, B00000001, B00000000, // F
4, 8, B00111110, B01000001, B01001001, B01111010, B00000000, // G
4, 8, B01111111, B00001000, B00001000, B01111111, B00000000, // H
3, 8, B01000001, B01111111, B01000001, B00000000, B00000000, // I
4, 8, B00110000, B01000000, B01000001, B00111111, B00000000, // J
4, 8, B01111111, B00001000, B00010100, B01100011, B00000000, // K
4, 8, B01111111, B01000000, B01000000, B01000000, B00000000, // L
5, 8, B01111111, B00000010, B00001100, B00000010, B01111111, // M
5, 8, B01111111, B00000100, B00001000, B00010000, B01111111, // N
4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // O
4, 8, B01111111, B00001001, B00001001, B00000110, B00000000, // P
4, 8, B00111110, B01000001, B01000001, B10111110, B00000000, // Q
4, 8, B01111111, B00001001, B00001001, B01110110, B00000000, // R
4, 8, B01000110, B01001001, B01001001, B00110010, B00000000, // S
5, 8, B00000001, B00000001, B01111111, B00000001, B00000001, // T
4, 8, B00111111, B01000000, B01000000, B00111111, B00000000, // U
5, 8, B00001111, B00110000, B01000000, B00110000, B00001111, // V
5, 8, B00111111, B01000000, B00111000, B01000000, B00111111, // W
5, 8, B01100011, B00010100, B00001000, B00010100, B01100011, // X
5, 8, B00000111, B00001000, B01110000, B00001000, B00000111, // Y
4, 8, B01100001, B01010001, B01001001, B01000111, B00000000, // Z
2, 8, B01111111, B01000001, B00000000, B00000000, B00000000, // [
4, 8, B00000001, B00000110, B00011000, B01100000, B00000000, // \ backslash
2, 8, B01000001, B01111111, B00000000, B00000000, B00000000, // ]
3, 8, B00000010, B00000001, B00000010, B00000000, B00000000, // hat
4, 8, B01000000, B01000000, B01000000, B01000000, B00000000, // _
2, 8, B00000001, B00000010, B00000000, B00000000, B00000000, // `
4, 8, B00100000, B01010100, B01010100, B01111000, B00000000, // a
4, 8, B01111111, B01000100, B01000100, B00111000, B00000000, // b
4, 8, B00111000, B01000100, B01000100, B00101000, B00000000, // c
4, 8, B00111000, B01000100, B01000100, B01111111, B00000000, // d
4, 8, B00111000, B01010100, B01010100, B00011000, B00000000, // e
3, 8, B00000100, B01111110, B00000101, B00000000, B00000000, // f
4, 8, B10011000, B10100100, B10100100, B01111000, B00000000, // g
4, 8, B01111111, B00000100, B00000100, B01111000, B00000000, // h
3, 8, B01000100, B01111101, B01000000, B00000000, B00000000, // i
4, 8, B01000000, B10000000, B10000100, B01111101, B00000000, // j
4, 8, B01111111, B00010000, B00101000, B01000100, B00000000, // k
3, 8, B01000001, B01111111, B01000000, B00000000, B00000000, // l
5, 8, B01111100, B00000100, B01111100, B00000100, B01111000, // m
4, 8, B01111100, B00000100, B00000100, B01111000, B00000000, // n
4, 8, B00111000, B01000100, B01000100, B00111000, B00000000, // o
4, 8, B11111100, B00100100, B00100100, B00011000, B00000000, // p
4, 8, B00011000, B00100100, B00100100, B11111100, B00000000, // q
4, 8, B01111100, B00001000, B00000100, B00000100, B00000000, // r
4, 8, B01001000, B01010100, B01010100, B00100100, B00000000, // s
3, 8, B00000100, B00111111, B01000100, B00000000, B00000000, // t
4, 8, B00111100, B01000000, B01000000, B01111100, B00000000, // u
5, 8, B00011100, B00100000, B01000000, B00100000, B00011100, // v
5, 8, B00111100, B01000000, B00111100, B01000000, B00111100, // w
5, 8, B01000100, B00101000, B00010000, B00101000, B01000100, // x
4, 8, B10011100, B10100000, B10100000, B01111100, B00000000, // y
3, 8, B01100100, B01010100, B01001100, B00000000, B00000000, // z
3, 8, B00001000, B00110110, B01000001, B00000000, B00000000, // {
1, 8, B01111111, B00000000, B00000000, B00000000, B00000000, // |
3, 8, B01000001, B00110110, B00001000, B00000000, B00000000, // }
4, 8, B00001000, B00000100, B00001000, B00000100, B00000000, // ~
};
int DIN = 12; // DIN pin of MAX7219 module
int CLK = 11; // CLK pin of MAX7219 module
int CS = 10; // CS pin of MAX7219 module
int maxInUse = 2;
MaxMatrix m(DIN, CS, CLK, maxInUse);
byte buffer[10];
char text[]= "AMAYRA FATIMA * FARAZ AHMAD * ARSHEEN ARIF * "; // Scrolling text
void setup() {
m.init(); // module initialize
m.setIntensity(4); // dot matix intensity 0-15
}
void loop() {
printStringWithShift(text, 250); // (text, scrolling speed)
}
// Display=the extracted characters with scrolling
void printCharWithShift(char c, int shift_speed) {
if (c < 32) return;
c -= 32;
memcpy_P(buffer, CH + 7 * c, 7);
m.writeSprite(32, 0, buffer);
m.setColumn(32 + buffer[0], 0);
for (int i = 0; i < buffer[0] + 1; i++)
{
delay(shift_speed);
m.shiftLeft(false, false);
}
}
// Extract the characters from the text string
void printStringWithShift(char* s, int shift_speed) {
while (*s != 0) {
printCharWithShift(*s, shift_speed);
s++;
}
}
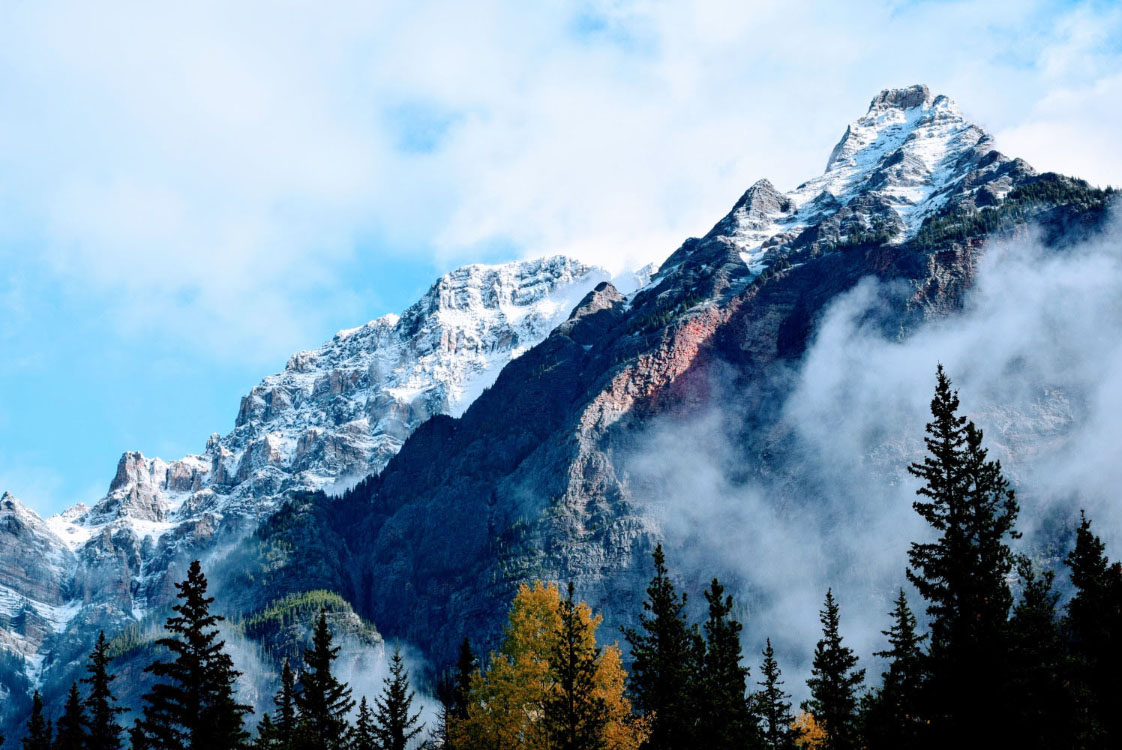
Thanks for Coding!
Home Automation System Under ₹ 1000
Google Voice Assistance + Actions on Google + Google Firebase + NodeMCU
A brief tutorial for a personal home automation device controlling 3 devices.
Developed by: Faraz Ahmad with lots of help from Areeb Jamal
Learner’s Level: Intermediate to Advanced
#InternetOfThings
Home Automation App (User’s Guide)
There are two modes of operation:
- OFF-LINE: Select this if you are controlling the appliances from within the home.
- ON-LINE: Select this if you are controlling the appliances from outside the home.
When OFF-LINE mode is selected, select the URL from the drop-down menu that appears on the device’s LED Screen.
Click the Button of each device to “Turn On” and Long Press that button to “Turn OFF“
Isse Pehle ki Bewafa Ho jaen- Ahmad Faraz
Iss Se Pehle Ki Bewafa Ho Jaaye
Kyun Na Aye Dost Hum Juda Ho Jaaye
Tu Bhi Hire Se Ban Gaya Patthar
Hum Bhi Kal Jane Kya Se Kya Ho Jaye
Hum Bhi Mazburiyon Ka Uzr Kare
Aur Kahin Aur Mubtalla Ho Jaye
Ishq Bhi Khel Hai Naseebon Ka
Khaak Ho Jaayege Kimeeyan Ho Jaaye
Ab Ke Gar Tu Mile To Hum Tujhse
Aise Lipte Teri Kabaa Ho Jaye
Bandgi Humne Chord Di Hai Faraz
Kya Kare Log Jab Khuda Ho Jaaye
Islanding and Black Start Exercise
Introduction
Electrical Grid System
All the energy transaction takes place through the Electrical Grid network which makes it the most important asset of the nation. Grid failure in 2012 (30-31 July, 2012) raised the need of strengthening the grid. The entire electrical grid system (to be referred to as “grid” from here onwards) is composed of numerous generators and loads. The stability of the grid thus remains a critical issue and hence it is necessary to ensure uninterrupted supply of electricity throughout. Failure of the grid is a Disaster in itself and it lead to the formation of a guidelines to manage this disaster which involves periodic monitoring and preparedness of the entire system to avoid and mitigate any such black-outs in the future.
Gourmet’s Travelogue of Srinagar & Gulmarg
گر فردوس بار رو ا زمین است ہمیں است-و ہمیں است-و ہمیں است
‘gar firdaus bar roo-e zameen ast, Hameen ast-o hameen ast-o hameen ast- Amir Khusrao
The couplet by the famous poet- Amir Khusrao means, “If there is a paradise on earth, It is this, it is this, it is this” and its truly said so. The beauty of the state is incoherent with the seasons and changes every day with the rising sun.
I present a brief Gourmet’s list for a short stay at Srinagar, the capital city of J&K. Continue reading “Gourmet’s Travelogue of Srinagar & Gulmarg”
Protected: Commercial and O&M Aspects of HPP-Concepts of Electricity Market
Roasted Dum Chicken
Inspired by the tender mutton recipe by one of my elders, i tried to give the Dum effect to roasted chicken. Its an easy task but this time I did one thing differently- marination.
So lets get started. Continue reading “Roasted Dum Chicken”